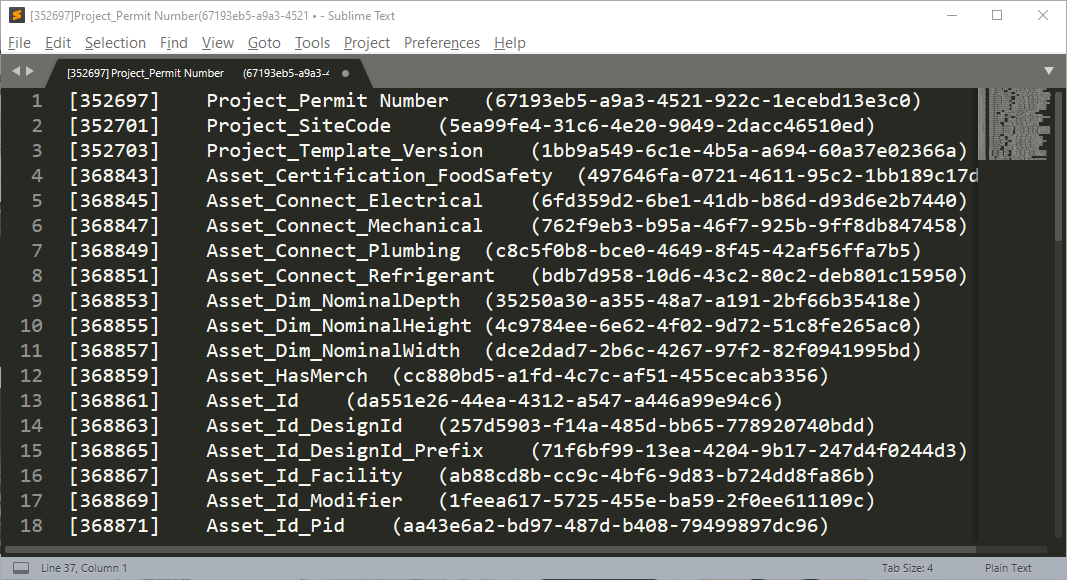
Programmatically Retrieve all Shared Parameters in a Project Model using The Revit API
A few months ago, I shared a post on OpenDefinery about a simple solution to fully purge Shared Parameters from a Revit project model. In that post, I shared my discovery that Shared Parameters have an Element ID just like any other model element, which means you can select the parameter through the Revit UI using the Select Model Elements by ID tool and simply hit the DELETE
key.
Building on that discovery, I wanted to share a code snippet of how to retrieve Shared Parameters in a project using the FilteredElementCollector class from the Revit API. If that sounds interesting, read on for a short code sample which demonstrates how to retrieve all Shared Parameters from a Revit project model.
Note that line 18 writes to the debug console, so if you’re planning to keep that line, you’ll need to add the statement using System.Diagnostics;
to your macro or add-in.
The output is a tab-delimited string formatted as: [Element ID
] [Parameter Name][Parameter GUID]\n
.
UIDocument uidoc = this.ActiveUIDocument; Document doc = uidoc.Document; List<Element> sharedParams = new List<Element>(); FilteredElementCollector collector = new FilteredElementCollector(doc) .WhereElementIsNotElementType(); // Filter elements for shared parameters only collector.OfClass(typeof(SharedParameterElement)); foreach(Element e in collector) { SharedParameterElement param = e as SharedParameterElement; Definition def = param.GetDefinition(); Debug.WriteLine("[" + e.Id + "]\t" + def.Name + "\t(" + param.GuidValue + ")"); }
This is just scratching the surface of the Revit add-in that I’m working on which connects OpenDefinery Shared Parameters to a Revit project model. Stay tuned to see how OpenDefinery is reinventing the way Shared Parameters are managed an implemented in Revit.
Hope this helps someone out there someday! Happy coding.