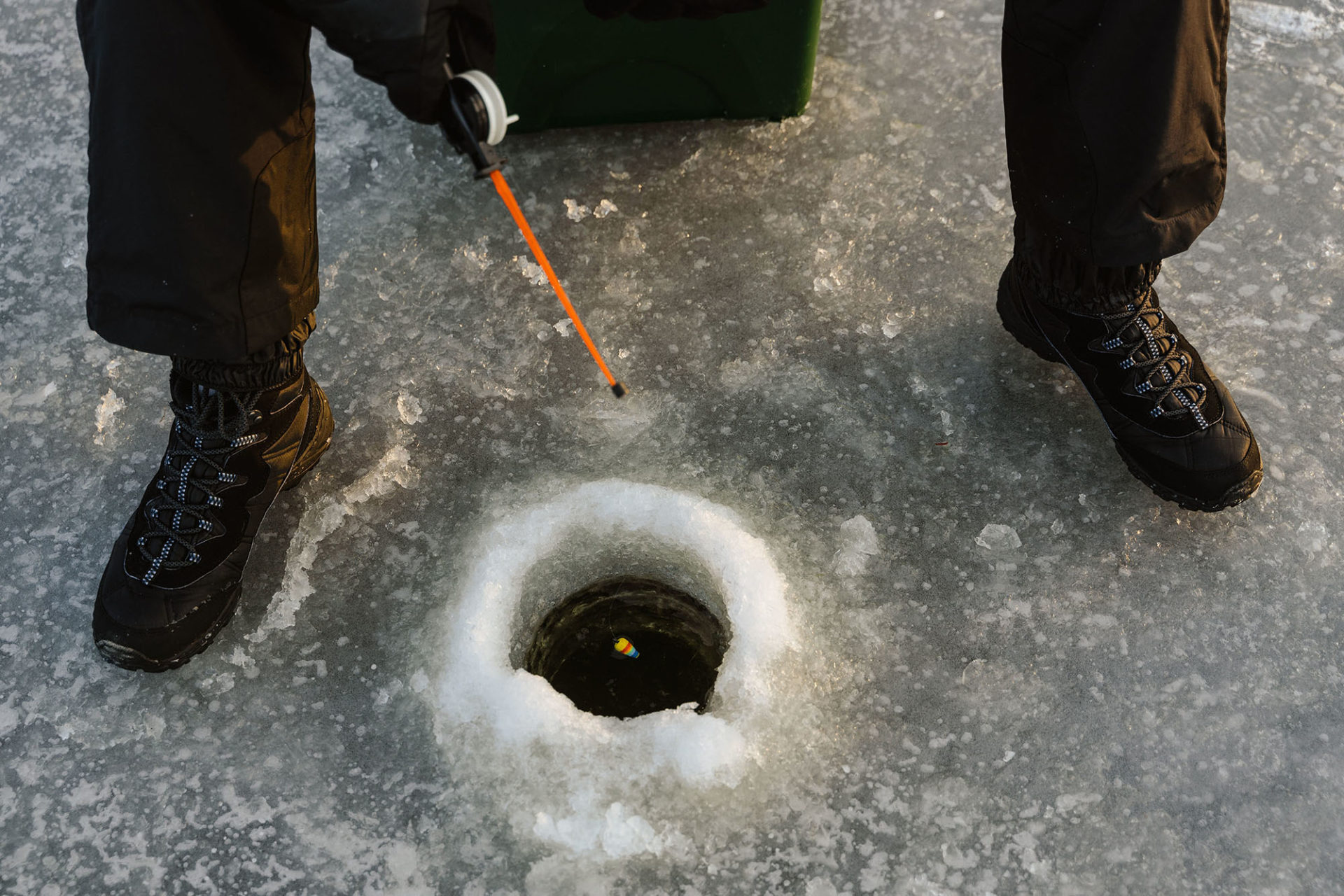
Retrieve Parameter Values Using the Revit API
When working with BIM data using the Revit API, one common step is retrieving parameter values from elements. Whether your goal is to extract or modify the data of an Element, reading parameter data programmatically is almost always going to be your first step. This is a relatively simple task, however it can be a little tricky for those who are new to the Revit API (or you’re like me and you simply keep forgetting). With that said, let’s review the three methods that I use religiously when working with parameter data using the Revit API.
Please don’t hesitate to drop me a comment if you have any questions or if you have any suggestions on how to improve my hacky, wannabe software developer methods.
Retrieve a Parameter Value by Name
public string GetParamValueByName(string name, Element e) { var paramValue = string.Empty; foreach (Parameter parameter in e.Parameters) { if (parameter.Definition.Name == name) { paramValue = parameter.AsString(); } } return paramValue; }
Assume that the Element e
which is passed to the method above is a selected model element (i.e., a family instance that the end user selected in a model). In this scenario, retrieving the parameter value is as simple as looping through the element’s Parameters property to find the parameter with the matching name.
Two more things…
- The parameter’s
Name
is a property is within the Definition property of the Parameter, and not a property of the parameter itself (as shown in line #7). - Look into the ParameterSet.ForwardIterator method. It seems to be a best practice when looping through Revit parameters, but I’m too lazy to learn how to use it. If you have any tips on using this method, please drop me a comment below!
Retrieve a Shared Parameter Value by GUID
public string GetParamValueByGuid(Guid guid, Element e) { var paramValue = string.Empty; foreach (Parameter parameter in e.Parameters) { if (parameter.IsShared) { if(parameter.GUID == guid) { paramValue = parameter.AsString(); } } } return paramValue; }
Shared parameters can be retrieved using the Parameter.Definition.Name as demonstrated in the previous example, however I always recommend to retrieve a shared parameter using its GUID. In my opinion this is an important distinction in best practices, so keep this in mind as your progress on your Revit API journey:
A model can have shared parameters with identical names, but never duplicate GUIDs.
In the example above, you can see that we are passing the parameter’s GUID to the method instead of the name.
Note: the GUID property is NOT a property of the Definition
class like the Name
example above, but rather the Parameter
class.
If you’re curious to learn more about the correct way to work with shared parameters in Revit, check out my side project: OpenDefinery.
<!– END SHAMELESS PLUG –>
Retrieving Type Parameters
foreach (Element element in pickedElements) { // Get the Family Type of the element for type parameters var typeId = element.GetTypeId(); var famSym = this.ActiveUIDocument.Document.GetElement(typeId) as FamilySymbol; if (famSym != null) { var assetId = GetParamValueByGuid( new Guid("7cc39847-53ae-495e-a8c6-1064be1da257"), famSym ); outputString += assetId + "\n"; } }
In Revit, parameters can be bound as either instance or type, which can make things tricky when accessing them via the Revit API. Earlier in this post, I mentioned that the Element e
represented a model element in which the end user selected. Now, if we are to get more technical, what this really means is that you have selected an instance of a family. Therefore, when you reference all Parameters of that Element, you are actually only accessing the instance parameters of a particular family instance.
To retrieve the type parameters of a family, you first need to retrieve the family type that the selected instance belongs to, and then retrieve the parameters of the family type object. To do this, you can see in line #5 above, we use the GetElement() method and pass the Element.Id returned from the GetTypeId() method in line #4.
Hope this helps.
I hope this helps someone out there someday. I know it will be a good copy-and-paste resource for me the next time I forget how to extract parameter values using the Revit API!